Creating a fully functional Jarvis using Dialogflow and Python requires a few steps. Here’s a high-level overview of the process:
1. Create a Dialogflow Agent: First, you need to create a Dialogflow agent and define its intents, entities, and responses. Intents represent the user’s intentions, and entities represent important variables that the user might mention. Responses are the actions that the agent takes in response to user input.
2. Set up a webhook in Dialogflow: A webhook is a URL that Dialogflow will call whenever a user input matches one of your intents. You can set up a webhook in Dialogflow by creating a fulfillment for your intents and specifying the URL of your Python script.
3. Create a Python Script: Your Python script will handle the incoming requests from Dialogflow and perform the appropriate actions. You’ll need to use a webhook framework like Flask or Django to create an endpoint that Dialogflow can call. Your script should parse the JSON payload sent by Dialogflow, extract the user’s intent and entities, and perform the appropriate actions.
4. Integrate External APIs: You can integrate external APIs such as weather API, news API, and other available APIs with your Python script to fetch information and respond to the user’s requests.
Here’s a sample code that demonstrates the implementation of a simple Jarvis using Dialogflow and Python.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(‘/webhook’, methods=[‘POST’])
def webhook():
req = request.get_json(force=True)
intent_name = req[“queryResult”][“intent”][“displayName”]
if intent_name == “greeting”:
response = {“fulfillmentText”: “Hi, how can I help you?”}
elif intent_name == “weather”:
city = req[“queryResult”][“parameters”][“geo-city”]
# Use external weather API to get the weather information for the city
# Formulate response based on weather information
response = {“fulfillmentText”: f”The weather in {city} is sunny today.”}
else:
response = {“fulfillmentText”: “I’m sorry, I didn’t understand your request.”}
return jsonify(response)
if __name__ == ‘__main__’:
app.run(debug=True)
This code defines a Flask endpoint that Dialogflow can call when a user sends a message. The code checks the user’s intent and entities and responds accordingly. The greeting
intent simply responds with a greeting message, while the weather
intent uses an external API to get the weather information for the specified city and returns the weather information to the user.
Note: The above code is just a sample implementation of a simple Jarvis using Dialogflow and Python. The actual implementation of a fully functional Jarvis requires much more than this, including handling multiple intents, integrating multiple APIs, and handling edge cases.
Working with a top-rated custom mobile app development company in Los Angeles ensures that your Jarvis-like AI app is developed with cutting-edge technology, offering a seamless and intelligent user experience.
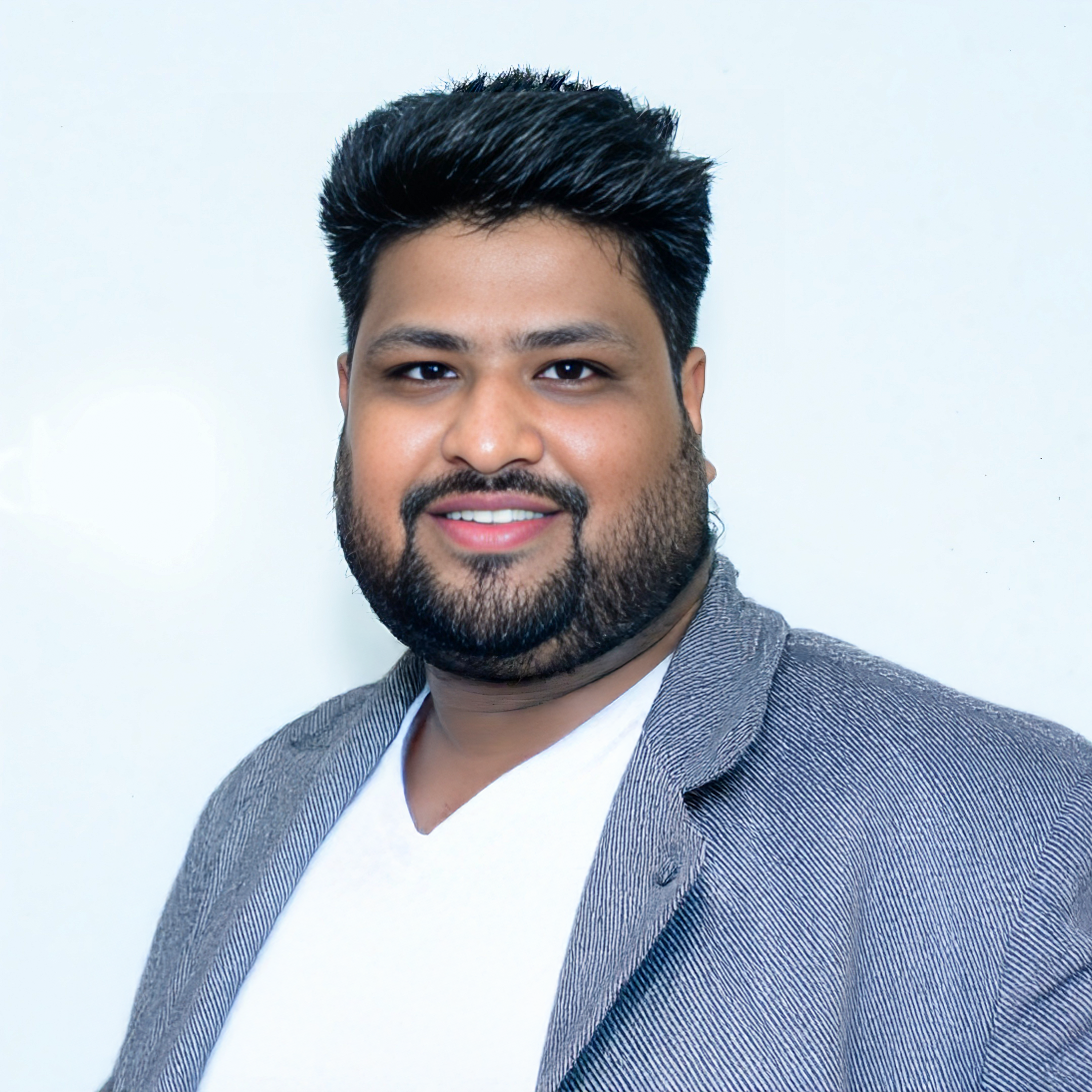
Hello All,
Aman Mishra has years of experience in the IT industry. His passion for helping people in all aspects of mobile app development. Therefore, He write several blogs that help the readers to get the appropriate information about mobile app development trends, technology, and many other aspects.In addition to providing mobile app development services in USA, he also provides maintenance & support services for businesses of all sizes. He tried to solve all their readers’ queries and ensure that the given information would be helpful for them.