There are several ways to skip a specific data point in Python, depending on the type of data structure you are working with. Here are some examples:
1. List comprehension:
If you have a list and want to skip a specific element, you can use a list comprehension. For example, to skip the element at index 2:
my_list = [1, 2, 3, 4, 5]
new_list = [x for i, x in enumerate(my_list) if i != 2]
This creates a new list without the element at index 2.
2. Conditional statement:
If you are iterating over a list or any iterable and want to skip a specific element based on a condition, you can use a conditional statement. For example, to skip any negative numbers in a list:
my_list = [-1, 2, -3, 4, -5]
for num in my_list:
if num < 0:
continue # skips the negative number
print(num)
This skips any negative number and continues iterating over the remaining elements.
3. Pandas Dataframe:
If you have a pandas dataframe and want to skip a specific row or column, you can use the .drop() method. For example, to drop the row with index 2:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
new_df = df.drop(2) # drops the row with index 2
This creates a new dataframe without the row with index 2. To drop a specific column, you can use the axis parameter:
new_df = df.drop('B', axis=1) # drops the column 'B'
This creates a new dataframe without the column ‘B’.
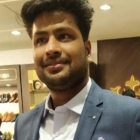
Hello All,
Aman Mishra has years of experience in the IT industry. His passion for helping people in all aspects of mobile app development. Therefore, He write several blogs that help the readers to get the appropriate information about mobile app development trends, technology, and many other aspects.In addition to providing mobile app development services in USA, he also provides maintenance & support services for businesses of all sizes. He tried to solve all their readers’ queries and ensure that the given information would be helpful for them.